Stringed 2 8

In the following examples, input and output are distinguished by the presence orabsence of prompts (>>> and …): to repeat the example, you must typeeverything after the prompt, when the prompt appears; lines that do not beginwith a prompt are output from the interpreter. Note that a secondary prompt on aline by itself in an example means you must type a blank line; this is used toend a multi-line command.
Many of the examples in this manual, even those entered at the interactiveprompt, include comments. Comments in Python start with the hash character,#
, and extend to the end of the physical line. A comment may appear at thestart of a line or following whitespace or code, but not within a stringliteral. A hash character within a string literal is just a hash character.Since comments are to clarify code and are not interpreted by Python, they maybe omitted when typing in examples.
MENDELSSOHN, Felix: String Quartets, Vol. 2 (New Zealand String Quartet) - String Quartets Nos. 2, 5 / Capriccio / Fugue The New Zealand String Quartet’s first Naxos recording of Mendelssohn’s string quartets ( 8.570001 ) was praised as ‘an auspicious start’ by Gramophone and ‘opulently recorded’ by Classic FM magazine.
- Music by Kevin MacLeod. Available under the Creative Commons Attribution 3.0 Unported license. Download link: https://incompetech.com/music/royalty-free/inde.
- Music by Kevin MacLeod. Available under the Creative Commons Attribution 3.0 Unported license. Download link: https://incompetech.com/music/royalty-free/inde.
- Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus id risus felis. Proin elit nulla, elementum sit amet diam rutrum, mollis venenatis felis. Nullam dapibus lacus justo, eget ullamcorper justo cursus ac. Aliquam lorem nulla, blandit id erat id, eleifend fermentum lorem.
- So I have compiled a list of manufacturers who make 8 string guitars. It may be useful to anyone shopping or curious about 8 strings. I'm doing a stor.
Some examples:
3.1. Using Python as a Calculator¶
Let’s try some simple Python commands. Start the interpreter and wait for theprimary prompt, >>>
. (It shouldn’t take long.)
3.1.1. Numbers¶
The interpreter acts as a simple calculator: you can type an expression at itand it will write the value. Expression syntax is straightforward: theoperators +
, -
, *
and /
work just like in most other languages(for example, Pascal or C); parentheses (()
) can be used for grouping.For example:
The integer numbers (e.g. 2
, 4
, 20
) have type int
,the ones with a fractional part (e.g. 5.0
, 1.6
) have typefloat
. We will see more about numeric types later in the tutorial.
The return type of a division (/
) operation depends on its operands. Ifboth operands are of type int
, floor division is performedand an int
is returned. If either operand is a float
,classic division is performed and a float
is returned. The //
operator is also provided for doing floor division no matter what theoperands are. The remainder can be calculated with the %
operator:
With Python, it is possible to use the **
operator to calculate powers 1:
The equal sign (=
) is used to assign a value to a variable. Afterwards, noresult is displayed before the next interactive prompt:
If a variable is not “defined” (assigned a value), trying to use it willgive you an error:
There is full support for floating point; operators with mixed type operandsconvert the integer operand to floating point:
In interactive mode, the last printed expression is assigned to the variable_
. This means that when you are using Python as a desk calculator, it issomewhat easier to continue calculations, for example:
This variable should be treated as read-only by the user. Don’t explicitlyassign a value to it — you would create an independent local variable with thesame name masking the built-in variable with its magic behavior.
In addition to int
and float
, Python supports other types ofnumbers, such as Decimal
and Fraction
.Python also has built-in support for complex numbers,and uses the j
or J
suffix to indicate the imaginary part(e.g. 3+5j
).
3.1.2. Strings¶
Besides numbers, Python can also manipulate strings, which can be expressedin several ways. They can be enclosed in single quotes ('..'
) ordouble quotes ('..'
) with the same result 2. can be usedto escape quotes:
In the interactive interpreter, the output string is enclosed in quotes andspecial characters are escaped with backslashes. While this might sometimeslook different from the input (the enclosing quotes could change), the twostrings are equivalent. The string is enclosed in double quotes ifthe string contains a single quote and no double quotes, otherwise it isenclosed in single quotes. The print
statement produces a morereadable output, by omitting the enclosing quotes and by printing escapedand special characters:
If you don’t want characters prefaced by to be interpreted asspecial characters, you can use raw strings by adding an
r
beforethe first quote:
String literals can span multiple lines. One way is using triple-quotes:''..''
or ''..''
. End of lines are automaticallyincluded in the string, but it’s possible to prevent this by adding a atthe end of the line. The following example:
produces the following output (note that the initial newline is not included):
Strings can be concatenated (glued together) with the +
operator, andrepeated with *
:
Two or more string literals (i.e. the ones enclosed between quotes) nextto each other are automatically concatenated.
This feature is particularly useful when you want to break long strings:
This only works with two literals though, not with variables or expressions:
If you want to concatenate variables or a variable and a literal, use +
:
Strings can be indexed (subscripted), with the first character having index 0.There is no separate character type; a character is simply a string of sizeone:
Indices may also be negative numbers, to start counting from the right:
Note that since -0 is the same as 0, negative indices start from -1.
In addition to indexing, slicing is also supported. While indexing is usedto obtain individual characters, slicing allows you to obtain a substring:
Note how the start is always included, and the end always excluded. Thismakes sure that s[:i]+s[i:]
is always equal to s
:
Slice indices have useful defaults; an omitted first index defaults to zero, anomitted second index defaults to the size of the string being sliced.
One way to remember how slices work is to think of the indices as pointingbetween characters, with the left edge of the first character numbered 0.Then the right edge of the last character of a string of n characters hasindex n, for example:
The first row of numbers gives the position of the indices 0…6 in the string;the second row gives the corresponding negative indices. The slice from i toj consists of all characters between the edges labeled i and j,respectively.
For non-negative indices, the length of a slice is the difference of theindices, if both are within bounds. For example, the length of word[1:3]
is2.
Attempting to use an index that is too large will result in an error:
However, out of range slice indexes are handled gracefully when used forslicing:
Python strings cannot be changed — they are immutable.Therefore, assigning to an indexed position in the string results in an error:
If you need a different string, you should create a new one:
The built-in function len()
returns the length of a string:
See also
Strings, and the Unicode strings described in the next section, areexamples of sequence types, and support the common operations supportedby such types.
Both strings and Unicode strings support a large number of methods forbasic transformations and searching.
Information about string formatting with str.format()
.
The old formatting operations invoked when strings and Unicode strings arethe left operand of the %
operator are described in more detail here.
3.1.3. Unicode Strings¶
Starting with Python 2.0 a new data type for storing text data is available tothe programmer: the Unicode object. It can be used to store and manipulateUnicode data (see http://www.unicode.org/) and integrates well with the existingstring objects, providing auto-conversions where necessary.
Unicode has the advantage of providing one ordinal for every character in everyscript used in modern and ancient texts. Previously, there were only 256possible ordinals for script characters. Texts were typically bound to a codepage which mapped the ordinals to script characters. This lead to very muchconfusion especially with respect to internationalization (usually written asi18n
— 'i'
+ 18 characters + 'n'
) of software. Unicode solvesthese problems by defining one code page for all scripts.
Creating Unicode strings in Python is just as simple as creating normalstrings:
The small 'u'
in front of the quote indicates that a Unicode string issupposed to be created. If you want to include special characters in the string,you can do so by using the Python Unicode-Escape encoding. The followingexample shows how:
The escape sequence u0020
indicates to insert the Unicode character withthe ordinal value 0x0020 (the space character) at the given position.
Other characters are interpreted by using their respective ordinal valuesdirectly as Unicode ordinals. If you have literal strings in the standardLatin-1 encoding that is used in many Western countries, you will find itconvenient that the lower 256 characters of Unicode are the same as the 256characters of Latin-1.
For experts, there is also a raw mode just like the one for normal strings. Youhave to prefix the opening quote with ‘ur’ to have Python use theRaw-Unicode-Escape encoding. It will only apply the above uXXXX
conversion if there is an uneven number of backslashes in front of the small‘u’.
The raw mode is most useful when you have to enter lots of backslashes, as canbe necessary in regular expressions.
Apart from these standard encodings, Python provides a whole set of other waysof creating Unicode strings on the basis of a known encoding.
The built-in function unicode()
provides access to all registered Unicodecodecs (COders and DECoders). Some of the more well known encodings which thesecodecs can convert are Latin-1, ASCII, UTF-8, and UTF-16. The latter twoare variable-length encodings that store each Unicode character in one or morebytes. The default encoding is normally set to ASCII, which passes throughcharacters in the range 0 to 127 and rejects any other characters with an error.When a Unicode string is printed, written to a file, or converted withstr()
, conversion takes place using this default encoding.
To convert a Unicode string into an 8-bit string using a specific encoding,Unicode objects provide an encode()
method that takes one argument, thename of the encoding. Lowercase names for encodings are preferred.
If you have data in a specific encoding and want to produce a correspondingUnicode string from it, you can use the unicode()
function with theencoding name as the second argument.
3.1.4. Lists¶
Python knows a number of compound data types, used to group together othervalues. The most versatile is the list, which can be written as a list ofcomma-separated values (items) between square brackets. Lists might containitems of different types, but usually the items all have the same type.
Like strings (and all other built-in sequence type), lists can beindexed and sliced:
All slice operations return a new list containing the requested elements. Thismeans that the following slice returns a new (shallow) copy of the list:
Lists also supports operations like concatenation:
Unlike strings, which are immutable, lists are a mutabletype, i.e. it is possible to change their content:
You can also add new items at the end of the list, by usingthe append()
method (we will see more about methods later):
Assignment to slices is also possible, and this can even change the size of thelist or clear it entirely:
The built-in function len()
also applies to lists:
It is possible to nest lists (create lists containing other lists), forexample:
3.2. First Steps Towards Programming¶
Of course, we can use Python for more complicated tasks than adding two and twotogether. For instance, we can write an initial sub-sequence of the Fibonacciseries as follows:
This example introduces several new features.
The first line contains a multiple assignment: the variables
a
andb
simultaneously get the new values 0 and 1. On the last line this is used again,demonstrating that the expressions on the right-hand side are all evaluatedfirst before any of the assignments take place. The right-hand side expressionsare evaluated from the left to the right.The
while
loop executes as long as the condition (here:b<10
)remains true. In Python, like in C, any non-zero integer value is true; zero isfalse. The condition may also be a string or list value, in fact any sequence;anything with a non-zero length is true, empty sequences are false. The testused in the example is a simple comparison. The standard comparison operatorsare written the same as in C:<
(less than),>
(greater than), (equal to),<=
(less than or equal to),>=
(greater than or equal to)and!=
(not equal to).The body of the loop is indented: indentation is Python’s way of groupingstatements. At the interactive prompt, you have to type a tab or space(s) foreach indented line. In practice you will prepare more complicated inputfor Python with a text editor; all decent text editors have an auto-indentfacility. When a compound statement is entered interactively, it must befollowed by a blank line to indicate completion (since the parser cannotguess when you have typed the last line). Note that each line within a basicblock must be indented by the same amount.
The
print
statement writes the value of the expression(s) it isgiven. It differs from just writing the expression you want to write (as we didearlier in the calculator examples) in the way it handles multiple expressionsand strings. Strings are printed without quotes, and a space is insertedbetween items, so you can format things nicely, like this:A trailing comma avoids the newline after the output:
Note that the interpreter inserts a newline before it prints the next prompt ifthe last line was not completed.
Footnotes
Since **
has higher precedence than -
, -3**2
will beinterpreted as -(3**2)
and thus result in -9
. To avoid thisand get 9
, you can use (-3)**2
.
Unlike other languages, special characters such as n
have thesame meaning with both single ('..'
) and double ('..'
) quotes.The only difference between the two is that within single quotes you don’tneed to escape '
(but you have to escape '
) and vice versa.
Source code:Lib/string.py
String constants¶
The constants defined in this module are:
string.
ascii_letters
¶The concatenation of the ascii_lowercase
and ascii_uppercase
constants described below. This value is not locale-dependent.
string.
ascii_lowercase
¶The lowercase letters 'abcdefghijklmnopqrstuvwxyz'
. This value is notlocale-dependent and will not change.
string.
ascii_uppercase
¶The uppercase letters 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
. This value is notlocale-dependent and will not change.
string.
digits
¶The string '0123456789'
.
string.
hexdigits
¶The string '0123456789abcdefABCDEF'
.
string.
octdigits
¶The string '01234567'
.
string.
punctuation
¶Stringed 2 80s
String of ASCII characters which are considered punctuation charactersin the C
locale: !'#$%&'()*+,-./:;<=>?@[]^_`{|}~
.
string.
printable
¶String of ASCII characters which are considered printable. This is acombination of digits
, ascii_letters
, punctuation
,and whitespace
.
string.
whitespace
¶A string containing all ASCII characters that are considered whitespace.This includes the characters space, tab, linefeed, return, formfeed, andvertical tab.
Custom String Formatting¶
The built-in string class provides the ability to do complex variablesubstitutions and value formatting via the format()
method described inPEP 3101. The Formatter
class in the string
module allowsyou to create and customize your own string formatting behaviors using the sameimplementation as the built-in format()
method.
string.
Formatter
¶The Formatter
class has the following public methods:
format
(format_string, /, *args, **kwargs)¶The primary API method. It takes a format string andan arbitrary set of positional and keyword arguments.It is just a wrapper that calls vformat()
.
Changed in version 3.7: A format string argument is now positional-only.
vformat
(format_string, args, kwargs)¶This function does the actual work of formatting. It is exposed as aseparate function for cases where you want to pass in a predefineddictionary of arguments, rather than unpacking and repacking thedictionary as individual arguments using the *args
and **kwargs
syntax. vformat()
does the work of breaking up the format stringinto character data and replacement fields. It calls the variousmethods described below.
In addition, the Formatter
defines a number of methods that areintended to be replaced by subclasses:
parse
(format_string)¶Loop over the format_string and return an iterable of tuples(literal_text, field_name, format_spec, conversion). This is usedby vformat()
to break the string into either literal text, orreplacement fields.
The values in the tuple conceptually represent a span of literal textfollowed by a single replacement field. If there is no literal text(which can happen if two replacement fields occur consecutively), thenliteral_text will be a zero-length string. If there is no replacementfield, then the values of field_name, format_spec and conversionwill be None
.
get_field
(field_name, args, kwargs)¶
Given field_name as returned by parse()
(see above), convert it toan object to be formatted. Returns a tuple (obj, used_key). The defaultversion takes strings of the form defined in PEP 3101, such as“0[name]” or “label.title”. args and kwargs are as passed in tovformat()
. The return value used_key has the same meaning as thekey parameter to get_value()
.
get_value
(key, args, kwargs)¶Retrieve a given field value. The key argument will be either aninteger or a string. If it is an integer, it represents the index of thepositional argument in args; if it is a string, then it represents anamed argument in kwargs.
The args parameter is set to the list of positional arguments tovformat()
, and the kwargs parameter is set to the dictionary ofkeyword arguments.
For compound field names, these functions are only called for the firstcomponent of the field name; subsequent components are handled throughnormal attribute and indexing operations.
So for example, the field expression ‘0.name’ would causeget_value()
to be called with a key argument of 0. The name
attribute will be looked up after get_value()
returns by calling thebuilt-in getattr()
function.
If the index or keyword refers to an item that does not exist, then anIndexError
or KeyError
should be raised.
check_unused_args
(used_args, args, kwargs)¶Implement checking for unused arguments if desired. The arguments to thisfunction is the set of all argument keys that were actually referred to inthe format string (integers for positional arguments, and strings fornamed arguments), and a reference to the args and kwargs that waspassed to vformat. The set of unused args can be calculated from theseparameters. check_unused_args()
is assumed to raise an exception ifthe check fails.
format_field
(value, format_spec)¶format_field()
simply calls the global format()
built-in. Themethod is provided so that subclasses can override it.
convert_field
(value, conversion)¶Converts the value (returned by get_field()
) given a conversion type(as in the tuple returned by the parse()
method). The defaultversion understands ‘s’ (str), ‘r’ (repr) and ‘a’ (ascii) conversiontypes.
Format String Syntax¶
The str.format()
method and the Formatter
class share the samesyntax for format strings (although in the case of Formatter
,subclasses can define their own format string syntax). The syntax isrelated to that of formatted string literals, butthere are differences. Mac keyboard and mouse.
Format strings contain “replacement fields” surrounded by curly braces {}
.Anything that is not contained in braces is considered literal text, which iscopied unchanged to the output. If you need to include a brace character in theliteral text, it can be escaped by doubling: {{
and }}
.
The grammar for a replacement field is as follows: Can i play fortnite on a macbook air.
In less formal terms, the replacement field can start with a field_name that specifiesthe object whose value is to be formatted and insertedinto the output instead of the replacement field.The field_name is optionally followed by a conversion field, which ispreceded by an exclamation point '!'
, and a format_spec, which is precededby a colon ':'
. These specify a non-default format for the replacement value.
See also the Format Specification Mini-Language section.
The field_name itself begins with an arg_name that is either a number or akeyword. If it’s a number, it refers to a positional argument, and if it’s a keyword,it refers to a named keyword argument. If the numerical arg_names in a format stringare 0, 1, 2, … in sequence, they can all be omitted (not just some)and the numbers 0, 1, 2, … will be automatically inserted in that order.Because arg_name is not quote-delimited, it is not possible to specify arbitrarydictionary keys (e.g., the strings '10'
or ':-]'
) within a format string.The arg_name can be followed by any number of index orattribute expressions. An expression of the form '.name'
selects the namedattribute using getattr()
, while an expression of the form '[index]'
does an index lookup using __getitem__()
.
Changed in version 3.1: The positional argument specifiers can be omitted for str.format()
,so '{}{}'.format(a,b)
is equivalent to '{0}{1}'.format(a,b)
.
Changed in version 3.4: The positional argument specifiers can be omitted for Formatter
.
Some simple format string examples:
The conversion field causes a type coercion before formatting. Normally, thejob of formatting a value is done by the __format__()
method of the valueitself. However, in some cases it is desirable to force a type to be formattedas a string, overriding its own definition of formatting. By converting thevalue to a string before calling __format__()
, the normal formatting logicis bypassed.
Three conversion flags are currently supported: '!s'
which calls str()
on the value, '!r'
which calls repr()
and '!a'
which callsascii()
.
Some examples:
The format_spec field contains a specification of how the value should bepresented, including such details as field width, alignment, padding, decimalprecision and so on. Each value type can define its own “formattingmini-language” or interpretation of the format_spec.
Most built-in types support a common formatting mini-language, which isdescribed in the next section.
A format_spec field can also include nested replacement fields within it.These nested replacement fields may contain a field name, conversion flagand format specification, but deeper nesting isnot allowed. The replacement fields within theformat_spec are substituted before the format_spec string is interpreted.This allows the formatting of a value to be dynamically specified.
See the Format examples section for some examples.
Format Specification Mini-Language¶
“Format specifications” are used within replacement fields contained within aformat string to define how individual values are presented (seeFormat String Syntax and Formatted string literals).They can also be passed directly to the built-informat()
function. Each formattable type may define how the formatspecification is to be interpreted.
Most built-in types implement the following options for format specifications,although some of the formatting options are only supported by the numeric types.
A general convention is that an empty format specification producesthe same result as if you had called str()
on the value. Anon-empty format specification typically modifies the result.
The general form of a standard format specifier is:
If a valid align value is specified, it can be preceded by a fillcharacter that can be any character and defaults to a space if omitted.It is not possible to use a literal curly brace (“{
” or “}
”) asthe fill character in a formatted string literal or when using the str.format()
method. However, it is possible to insert a curly bracewith a nested replacement field. This limitation doesn’taffect the format()
function.
The meaning of the various alignment options is as follows:
Option | Meaning |
---|---|
| Forces the field to be left-aligned within the availablespace (this is the default for most objects). |
| Forces the field to be right-aligned within theavailable space (this is the default for numbers). |
| Forces the padding to be placed after the sign (if any)but before the digits. This is used for printing fieldsin the form ‘+000000120’. This alignment option is onlyvalid for numeric types. It becomes the default when ‘0’immediately precedes the field width. |
| Forces the field to be centered within the availablespace. |
Note that unless a minimum field width is defined, the field width will alwaysbe the same size as the data to fill it, so that the alignment option has nomeaning in this case.
The sign option is only valid for number types, and can be one of thefollowing:
Option | Meaning |
---|---|
| indicates that a sign should be used for bothpositive as well as negative numbers. |
| indicates that a sign should be used only for negativenumbers (this is the default behavior). |
space | indicates that a leading space should be used onpositive numbers, and a minus sign on negative numbers. |
The '#'
option causes the “alternate form” to be used for theconversion. The alternate form is defined differently for differenttypes. This option is only valid for integer, float, complex andDecimal types. For integers, when binary, octal, or hexadecimal outputis used, this option adds the prefix respective '0b'
, '0o'
, or'0x'
to the output value. For floats, complex and Decimal thealternate form causes the result of the conversion to always contain adecimal-point character, even if no digits follow it. Normally, adecimal-point character appears in the result of these conversionsonly if a digit follows it. In addition, for 'g'
and 'G'
conversions, trailing zeros are not removed from the result.
The ','
option signals the use of a comma for a thousands separator.For a locale aware separator, use the 'n'
integer presentation typeinstead.
Changed in version 3.1: Added the ','
option (see also PEP 378).
The '_'
option signals the use of an underscore for a thousandsseparator for floating point presentation types and for integerpresentation type 'd'
. For integer presentation types 'b'
,'o'
, 'x'
, and 'X'
, underscores will be inserted every 4digits. For other presentation types, specifying this option is anerror.
Changed in version 3.6: Added the '_'
option (see also PEP 515).
width is a decimal integer defining the minimum total field width,including any prefixes, separators, and other formatting characters.If not specified, then the field width will be determined by the content.
When no explicit alignment is given, preceding the width field by a zero('0'
) character enablessign-aware zero-padding for numeric types. This is equivalent to a fillcharacter of '0'
with an alignment type of '='
.
The precision is a decimal number indicating how many digits should bedisplayed after the decimal point for a floating point value formatted with'f'
and 'F'
, or before and after the decimal point for a floating pointvalue formatted with 'g'
or 'G'
. For non-number types the fieldindicates the maximum field size - in other words, how many characters will beused from the field content. The precision is not allowed for integer values.
Finally, the type determines how the data should be presented.
The available string presentation types are:
Type Arcanum of steamworks and magick obscura download free.zip. | Meaning |
---|---|
| String format. This is the default type for strings andmay be omitted. |
None | The same as |
The available integer presentation types are:
Type | Meaning |
---|---|
| Binary format. Outputs the number in base 2. |
| Character. Converts the integer to the correspondingunicode character before printing. |
| Decimal Integer. Outputs the number in base 10. |
| Octal format. Outputs the number in base 8. |
| Hex format. Outputs the number in base 16, usinglower-case letters for the digits above 9. |
| Hex format. Outputs the number in base 16, usingupper-case letters for the digits above 9. |
| Number. This is the same as |
None | The same as |
In addition to the above presentation types, integers can be formattedwith the floating point presentation types listed below (except'n'
and None
). When doing so, float()
is used to convert theinteger to a floating point number before formatting.
The available presentation types for floating point and decimal values are:
Type | Meaning |
---|---|
| Exponent notation. Prints the number in scientificnotation using the letter ‘e’ to indicate the exponent.The default precision is |
| Exponent notation. Same as |
| Fixed-point notation. Displays the number as afixed-point number. The default precision is |
| Fixed-point notation. Same as |
| General format. For a given precision The precise rules are as follows: suppose that theresult formatted with presentation type Positive and negative infinity, positive and negativezero, and nans, are formatted as A precision of |
| General format. Same as |
| Number. This is the same as |
| Percentage. Multiplies the number by 100 and displaysin fixed ( |
None | Similar to |
Format examples¶
This section contains examples of the str.format()
syntax andcomparison with the old %
-formatting.
In most of the cases the syntax is similar to the old %
-formatting, with theaddition of the {}
and with :
used instead of %
.For example, '%03.2f'
can be translated to '{:03.2f}'
.
The new format syntax also supports new and different options, shown in thefollowing examples.
Accessing arguments by position:
Accessing arguments by name:
Accessing arguments’ attributes:
Accessing arguments’ items:
Replacing %s
and %r
:
Aligning the text and specifying a width:
Replacing %+f
, %-f
, and %f
and specifying a sign:
Replacing %x
and %o
and converting the value to different bases:
Using the comma as a thousands separator:
Expressing a percentage:
Using type-specific formatting:
Nesting arguments and more complex examples:
Template strings¶
Template strings provide simpler string substitutions as described inPEP 292. A primary use case for template strings is forinternationalization (i18n) since in that context, the simpler syntax andfunctionality makes it easier to translate than other built-in stringformatting facilities in Python. As an example of a library built on templatestrings for i18n, see theflufl.i18n package.
Template strings support $
-based substitutions, using the following rules:
$$
is an escape; it is replaced with a single$
.$identifier
names a substitution placeholder matching a mapping key of'identifier'
. By default,'identifier'
is restricted to anycase-insensitive ASCII alphanumeric string (including underscores) thatstarts with an underscore or ASCII letter. The first non-identifiercharacter after the$
character terminates this placeholderspecification.${identifier}
is equivalent to$identifier
. It is required whenvalid identifier characters follow the placeholder but are not part of theplaceholder, such as'${noun}ification'
.
Any other appearance of $
in the string will result in a ValueError
being raised.
The string
module provides a Template
class that implementsthese rules. The methods of Template
are:
string.
Template
(template)¶The constructor takes a single argument which is the template string.
substitute
(mapping={}, /, **kwds)¶Performs the template substitution, returning a new string. mapping isany dictionary-like object with keys that match the placeholders in thetemplate. Alternatively, you can provide keyword arguments, where thekeywords are the placeholders. When both mapping and kwds are givenand there are duplicates, the placeholders from kwds take precedence.
safe_substitute
(mapping={}, /, **kwds)¶Like substitute()
, except that if placeholders are missing frommapping and kwds, instead of raising a KeyError
exception, theoriginal placeholder will appear in the resulting string intact. Also,unlike with substitute()
, any other appearances of the $
willsimply return $
instead of raising ValueError
.
While other exceptions may still occur, this method is called “safe”because it always tries to return a usable string instead ofraising an exception. In another sense, safe_substitute()
may beanything other than safe, since it will silently ignore malformedtemplates containing dangling delimiters, unmatched braces, orplaceholders that are not valid Python identifiers.
Template
instances also provide one public data attribute:
template
¶This is the object passed to the constructor’s template argument. Ingeneral, you shouldn’t change it, but read-only access is not enforced.
Here is an example of how to use a Template:
Advanced usage: you can derive subclasses of Template
to customizethe placeholder syntax, delimiter character, or the entire regular expressionused to parse template strings. To do this, you can override these classattributes:
delimiter – This is the literal string describing a placeholderintroducing delimiter. The default value is
$
. Note that this shouldnot be a regular expression, as the implementation will callre.escape()
on this string as needed. Note further that you cannotchange the delimiter after class creation (i.e. a different delimiter mustbe set in the subclass’s class namespace).idpattern – This is the regular expression describing the pattern fornon-braced placeholders. The default value is the regular expression
(?a:[_a-z][_a-z0-9]*)
. If this is given and braceidpattern isNone
this pattern will also apply to braced placeholders.Note
Since default flags is
re.IGNORECASE
, pattern[a-z]
can matchwith some non-ASCII characters. That’s why we use the locala
flaghere.Changed in version 3.7: braceidpattern can be used to define separate patterns used inside andoutside the braces.
braceidpattern – This is like idpattern but describes the pattern forbraced placeholders. Defaults to
None
which means to fall back toidpattern (i.e. the same pattern is used both inside and outside braces).If given, this allows you to define different patterns for braced andunbraced placeholders.flags – The regular expression flags that will be applied when compilingthe regular expression used for recognizing substitutions. The default valueis
re.IGNORECASE
. Note thatre.VERBOSE
will always be added to theflags, so custom idpatterns must follow conventions for verbose regularexpressions.New in version 3.2.
Alternatively, you can provide the entire regular expression pattern byoverriding the class attribute pattern. If you do this, the value must be aregular expression object with four named capturing groups. The capturinggroups correspond to the rules given above, along with the invalid placeholderrule:
escaped – This group matches the escape sequence, e.g.
$$
, in thedefault pattern.named – This group matches the unbraced placeholder name; it should notinclude the delimiter in capturing group.
braced – This group matches the brace enclosed placeholder name; it shouldnot include either the delimiter or braces in the capturing group.
invalid – This group matches any other delimiter pattern (usually a singledelimiter), and it should appear last in the regular expression.
Helper functions¶
string.
capwords
(s, sep=None)¶Split the argument into words using str.split()
, capitalize each wordusing str.capitalize()
, and join the capitalized words usingstr.join()
. If the optional second argument sep is absentor None
, runs of whitespace characters are replaced by a single spaceand leading and trailing whitespace are removed, otherwise sep is used tosplit and join the words.
.. Word Origin fem. of shemini, qv. Sheminith Feminine of shmiyniy; probably an
eight-stringed lyre -- Sheminith. see HEBREW shmiyniy. 8066, 8067. ..
/hebrew/8067.htm - 5k
Eight-stringed. Eightieth, Eight-stringed. Eighty . Multi-Version
Concordance Eight-stringed (3 Occurrences). 1 Chronicles ..
/e/eight-stringed.htm - 7k
Lyre (32 Occurrences)
.. 1 Chronicles 15:21 and Mattithiah, and Eliphelehu, and Mikneiah, and Obed-Edom,
and Jeiel, and Azaziah, with harps tuned to the eight-stringed lyre, to lead. ..
/l/lyre.htm - 15k
Musician (112 Occurrences)
.. Psalms 6:1 For the Chief Musician; on stringed instruments, upon the eight-stringed
lyre. .. Psalms 12:1 For the Chief Musician; upon an eight-stringed lyre. ..
/m/musician.htm - 39k
Choirmaster (55 Occurrences)
.. Psalms 6:1 For the Chief Musician; on stringed instruments, upon the eight-stringed
lyre. .. Psalms 12:1 For the Chief Musician; upon an eight-stringed lyre. ..
/c/choirmaster.htm - 21k
Psalm (213 Occurrences)
.. Psalms 6:1 For the Chief Musician; on stringed instruments, upon the eight-stringed
lyre. .. Psalms 12:1 For the Chief Musician; upon an eight-stringed lyre. ..
/p/psalm.htm - 37k
Eighty (36 Occurrences)
/e/eighty.htm - 17k
Vanished (13 Occurrences)
.. (See RSV). Psalms 12:1 For the Chief Musician; upon an eight-stringed lyre.
A Psalm of David. Help, Yahweh; for the godly man ceases. ..
/v/vanished.htm - 10k
O'bed-e'dom (14 Occurrences)
.. 1 Chronicles 15:21 and Mattithiah, and Eliphelehu, and Mikneiah, and Obed-Edom,
and Jeiel, and Azaziah, with harps tuned to the eight-stringed lyre, to lead. ..
/o/o'bed-e'dom.htm - 10k
Je-i'el (11 Occurrences)
.. 1 Chronicles 15:21 and Mattithiah, and Eliphelehu, and Mikneiah, and Obed-Edom,
and Jeiel, and Azaziah, with harps tuned to the eight-stringed lyre, to lead. ..
/j/je-i'el.htm - 9k
Jeiel (13 Occurrences)
.. 1 Chronicles 15:21 and Mattithiah, and Eliphelehu, and Mikneiah, and Obed-Edom,
and Jeiel, and Azaziah, with harps tuned to the eight-stringed lyre, to lead. ..
/j/jeiel.htm - 13k
What is Hanukkah? Should a Christian celebrate Hanukkah (Christmaskah)? | GotQuestions.org
What is the significance of the Negev in the Bible? | GotQuestions.org
Bible Concordance • Bible Dictionary • Bible Encyclopedia • Topical Bible • Bible Thesuarus

Screenflow 7 3 – Create Screen Recordings Recorder

- Screenflow Software
- Screenflow 7 3 – Create Screen Recordings Recorder Download
- Screenflow Download Windows
Recording a screen used to be a real challenge.
You had to either use complicated tools or pay a software expert to get it done, which wasn’t always feasible.
The ScreenFlow 2018 License Key Free Download can record any type of the screen, the entire check. Although taking your video camera, iPad or iPhone, multi-funnel audio front. Along with your computer audio all concurrently. Then polish it with pros-level animations. Video motions, changes, and audio or video for incredible searching results. Camtasia: One of the best video editing software is undoubtedly Camtasia. This software was.
ScreenFlow: If you’re using a Mac and $ 199 Camtasia seems like a bit too much, you can contact ScreenFlow – an excellent desktop recording software that allows you to record your macOS desktop, webcam, and record both input and output sounds. This software also includes a simple editor that will enable you to mount the recorded videos, cut.
Fortunately, the user-friendliness of screen recording software has significantly improved over the years. Nowadays, you can create professional-looking tutorials with minimal effort.
Besides tutorials, there are several other reasons why you might want to record your screen.
If you do any kind of product demonstrations, need or offer tech support, or use video calling, using the default screen capture of your computer won’t be good enough to capture high-quality footage.
Whether you want a more precise way to record the entire screen or if you want to capture a specific portion, a high-quality screen recorder will serve the purpose.
In this article, you’ll learn about:
- What screen recording software is
- The features you should look for in screen recording software
- The difference between desktop and online screen recorder
- The 10 best screen recording tools in existence
Let’s go.
Don’t wait for someone else to do it. Hire yourself and start calling the shots.
Post Contents
- Top 10 Screen Recorder Tools for 2020
What Is Screen Recording Software?
Screen recording software can record the entirety (or portions) of your computer or mobile screen.
The recording can include everything from your taps and cursor movements to typing a URL into your browser to help people learn what to do and how to do it.
Audio narration is available, and sometimes annotation is also offered as part of a tool’s package.
However, no two screen recording tools are alike. Some screen recording tools can’t save the recorded footage for various formats or export it to popular video platforms like YouTube directly.
Others may have the capability to do all of that, but might be missing a feature or two. For example, not every screen recording application comes with an HD screen recorder.
As such, you should pay attention to its capabilities before downloading or installing screen recording software on your device.
It’s also crucial to evaluate your own recording needs. Perhaps you don’t need to record in HD. Maybe you’ve recently purchased video hosting and can do without a YouTube export option. The best screen recorder for you will be the one that meets your specific needs.
What Is a Good Screen Recorder?
Our compilation of screen recording tools ranges from browser-based software to minimalistic desktop apps. Each of the picks meets the following basic criteria:
- Is easy-to-use
- Records the entire screen, a particular area, or one window
- Lets users annotate the recorded footage
- Includes system and microphone audio
- Captures footage from external sources
- Has built-in export options
It’s also worth mentioning that many of the software options come with HD recording capabilities. However, it’s important to know that HD files take a lot of time to upload. If you’re looking to quickly uploaded standard-quality videos on the web, HD is not a must-have.
Desktop Screen Recorder vs. Online Screen Recorder
Offline functionality is the key difference between the two types.
While desktop screen recorders can work without an active connection, online screen recorders require you to have stable internet.
This is why an online screen recorder isn’t the best of options when it comes to capturing gameplay. However, if you’re only looking to make a tutorial, they should work just fine.
Top 10 Screen Recorder Tools for 2020
Here’s our list of the best screen recorder tools on the market.
Because it has options for all of the major operating systems, you can be sure to find the best screen recorder for Android, iOS, Mac, Linux and Windows operating systems.
Quick note: The list includes both paid and free options, so make sure to evaluate your needs before downloading any software.
1. OBS Studio
OBS or Open Broadcaster Software is a free screen video recorder that offers both recording and streaming in high definition, with no limits on the length of your videos.
The desktop software allows you to choose the part of the screen you want to capture, but it also gives an option to record the entire screen.
https://lbkjw.over-blog.com/2020/12/how-to-use-apps-on-mac.html. Customizable hotkeys help control the recording, and an audio mixer allows you to produce videos with smoothly recorded sound.
Apart from the intuitive screencasting features, OBS is equipped with live-streaming capabilities that you won’t find in the default screen recorders of major operating systems.
You can stream live on YouTube Gaming and Twitch, save projects, and convert your footage in FLV format.
All of this makes OBS one of the best free screen recording software options to try in 2020.
Price: Free
Supported OS: Linux, Mac, Windows
Best for: Professional live streaming to Mixer, YouTube or Twitch
2. Apowersoft Unlimited
This is a suite of software applications that includes screen recorders for Android, iOS, Mac and Windows.
It allows you to wirelessly cast an Android device or iPhone to your PC’s screen and use the desktop recorder to capture your PC audio, mobile device, microphone, and computer’s webcam simultaneously.
The desktop screen recorder offers multiple recording modes, including full screen, custom area, and more, and it also lets users annotate on the recording in real-time. You can apply callouts, lines, text, and more without needing to pause.
With the help of its intuitive, mobile-specific apps, you just have to set your computer and smartphone under the same WiFi network and begin casting.
Mac and Windows users can directly use the desktop screen recorder to record all kinds of on-screen activities.
Price: $59.95 per year
Supported OS: Android, iOS, Windows, Mac
Best for: Demonstrating how to use software that has both a desktop and a mobile version
3. Screencast-O-Matic
Screencast-O-Matic is an online screen recorder and video editor that supports both webcam and screen recordings.
While the application doesn’t let you record in HD, it does have options to record in 800×600, 640×480 and other standard definitions, which makes it ideal for recording and uploading quick videos.
It also assists users in zooming, drawing, and adding overlays on the video files.
Plus, you can share the recording to YouTube without having to download any desktop app.
Screencast-O-Matic also has additional features including options for editing computer audio and synchronization of recording with video footage.
Keep in mind that most of the editing features are offered in the paid version of the app. However, you can use the free version to upload content to YouTube.
Price: Free or $1.50 per month
Supported OS: Windows, Mac, Chromebook, iOS
Best for: Making quick tutorials to share with friends, customers or online communities
4. AceThinker
AceThinker is a web-based laptop screen recorder that is easy to set up and use.
You can use it to record a specific screen portion or the whole screen, create real-time edits for an attention-worthy recording, and capture audio from your mic and computer simultaneously.
The software lets you convert and save your recordings in all renowned video formats, including MP4, MOV and API.
And you can also use AceThinker to directly upload your recordings to Dropbox, Google Drive, or YouTube.
All of these features are available in the free version of the app, but you can also install the PRO version to gain access to additional capabilities.
A standout feature of the PRO version is that it lets you create scheduled tasks for screen recordings.
If you want to record an ongoing live stream in the afternoon, but you don’t have the internet at work, you can install this screen capture tool on your home computer, create a schedule and let it record the screen automatically.
Price: Free or $39.95
Supported OS: Mac, Windows
Best for: Recording soccer matches, stock trends, etc.
5. ScreenFlow
If you’ve been searching for a Mac screen recorder with audio capabilities, then you’d love ScreenFlow.
Its range of features includes the options to record your Mac at retina resolution, group video components and settings, and add background audio to the recorded footage.
You’d also love the “Styles” and “Templates” tools that come as part of the software.
Styles lets you save visual/audio settings for certain elements like drop shadows or color filters for a recorded segment.
Templates, on the other hand, lets users arrange, insert and configure groups of annotations in advance, such as text placeholders and outro segments.
Those who subscribe to the Super Pak version of ScreenFlow (a more expensive plan) also get access to over 500,000 pieces of unique images and audio clips to use in all of their videos.
So if you’re looking to make the most of your screen recordings, ScreenFlow is unlikely to disappoint.
Price: $129
Supported OS: Mac, iOS
Best for: Making high-quality retina videos
6. Screencastify
Screencastify is another screen recording application that works via Google Chrome.
Whether you’re a seasoned or a novice video creator, you’ll find that Screencastify offers all of the important options.
You can use it to capture your webcam, entire screen or tab only, as well as narrate to your microphone.
It also presents you with annotation tools that help keep your viewers focus on what’s critical. Click highlighting, drawing pen tool, and mouse spotlight are all included.
And once you’re recorded the screen, Screencastify will do the heavy lifting for you by autosaving the video to your Google Drive.
Like many of the best screen recording software options, Screencastify gives you the option to upload to YouTube, as well as export the recording as an animated GIF, MP3, or MP4.
Price: Free
Supported OS: Mac, Windows, Linux
Best for: Recording software demos and creating solution videos
7. Bandicam
Bandicam is a robust screen recording application that supports screen and gameplay capturing.
It utilizes a high compression ratio without compromising video quality. Bandicam makes it possible to capture 4K ultra-high-definition videos at 120 frames per second.
Another noteworthy feature is Bandicam’s device recording capability. By getting a separate capture card, you can record videos from IPTV, HDTV, APPLE TV, smartphone, PlayStation and Xbox.
In addition, Bandicam lets users capture screenshots in JPEG, PNG and BMP formats.
Essentially, you can use Bandicam to record just about anything, including your entire computer screen, Excel spreadsheets, web browser, Powerpoint presentations, and more.

Its free version places a watermark on the videos, so you need to become a paid user to utilize its full potential.
Price: Free or $39.99
Supported OS: Windows
Best for: Balancing the depth of video recordings
8. Filmora Scrn
Filmora Scrn lets you capture gameplay, webcam, computer screen and audio simultaneously without placing any limits on the duration of the video.
It gives you the option to record in 15-120 frames per second. Higher FPS is ideal for recording gameplay with loads of action.
The software also lets you add annotations like text to improve the viewing experience for your audience. Also, you can modify the size, color and shape of your cursor to make it look unique.
With Filmora Scrn, you don’t need a separate video editing tool because the screen recording software itself boasts a wide range of editing tools to help refine your video recordings.
It can import from over 50 file formats, and you also get the option to export your recordings in multiple formats as per your requirement.
Price: Free or $29.99
Supported OS: Windows, Mac
Best for: Recording screen from two devices simultaneously
9. Camtasia
TechSmith’s Camtasia makes it simple to capture and make professional-looking videos on your PC.
You can record both audio and video from a desktop or iOS device, and also capture your webcam to infuse a personal element to your recording.
Its built-in video editing tool is where Camtasia shines. A good selection of stock images along with click-and-drag effects is offered in the sidebar of the tool. Users can create outro and intro segments by dropping them into their recordings in a matter of seconds.
You can also insert zoom out, zoom in, and pan animations into your footage, and use transitions between slides and scenes to enhance the flow of your videos.
The screen recording software also lets you import or record PowerPoint pages directly into its environment. Grab attention in your presentations by adding eye-catching recordings, titles, and more.
Price: Free or $249
Supported OS: Windows, Mac, iOS
Best for: Adding a professional touch to captured videos
10. ShareX
Istat menus 5 3 1 download free download. This open-source screen recording software is ideal for capturing videos.
It doesn’t place watermarks or duration limits on your recordings, and you also get the option to save your file as a GIF instead of a video.
The video file sharing features are ShareX’s biggest advantage, because it’s easy to upload recordings directly to YouTube and other platforms.
Enhancing screenshots you capture with this tool doesn’t require much effort either. A built-in photo editor lets you freely customize still photos in any way you want.
Not only is it a brilliant screenshot and screen capture tool, but it can also capture and reveal text via OCR, record a whole scrolling webpage, and even record your screen according to a schedule.

Price: Free
Supported OS: Linux, Windows
Best for: Recording screens of Linux devices
Conclusion
Screen recording software can serve a variety of purposes, and the best ones don’t necessarily need to come at a price.
Based on your individual needs and the operating system you’re using, you may be able to achieve your objectives with a free screen recorder.
And if you’re looking to add additional power or use professional editing tools, you can always sign up to a paid option.
Hopefully, one of these screen recording programs will fulfill your requirements, whether you’re creating a tutorial for YouTube or just sharing a gaming achievement with your peers.
Do you use any awesome screen recording software that we missed? Let us know by dropping a comment below.
Want to Learn More?
In this post, we will see 5 best software to record PC screen with audio and webcam. For multiple reasons, you may need to record your computer screen, do advanced edits, and do any number of things without much effort. Our Windows computers do not come with a program that allows us to do this, but fortunately, there are other developers in charge of making our lives much easier.
If you are looking for a program to record the computer screen, here is a list of the best screen recorders for Windows, Mac OSX, Web, or even Linux operating systems. With them, you will enjoy hundreds of great and extremely useful functions, perfect for making video tutorials, recording steps or instructions of something you are teaching, showing a friend how you can use another program, and even creating and producing spectacular videos for your channel from YouTube.
Also Check:
Let’s get started with the software that can be used to record PC screen with ease.
Camtasia:
One of the best video editing software is undoubtedly Camtasia. This software was designed by Techsmith to compete with other more advanced ones such as Adobe Premiere or Sony Vegas, although not with the power of the latter. Creativemarket pro light leaks photoshop action download free. In any case, the advantages that this application provides us are many. You can make video edits, supporting high definition formats and with an exceptionally nice rendering system.
Besides, you will record your computer screen, taking advantage of the monitor’s resolution to capture all its characteristics. You can configure the outgoing sound so that the background music of the operating system is heard. However, it is also compatible with connected microphones, in case you want to do a good tutorial and need to narrate in real-time.
Available for Windows and macOS.
Screenflow Software
ScreenFlow:
If you’re using a Mac and $ 199 Camtasia seems like a bit too much, you can contact ScreenFlow – an excellent desktop recording software that allows you to record your macOS desktop, webcam, and record both input and output sounds. This software also includes a simple editor that will enable you to mount the recorded videos, cut, and edit various effects.
It is probably the best software of its kind available on the Apple platform. It is only available for MAC OSX, and although you may come across websites that try to sell ScreenFlow for Windows, they are deceptive websites, so be careful with fraudulent sites.
Available for macOS.
TinyTake:
There are those who record the screen to make presentations that explain a program’s functionality or organize a course. TinyTake is very different from the previous programs because it is based on allowing you to make a slide show, with a fairly good capture tool. Most importantly, it is free to download and use.
You can record up to 2 hours without interruption, in case it is an intensive one. Although it is advisable to make short videos to make it more synthesized.
Available for Windows.
RecordCast:
There are those who hate having to install programs and software on the computer. If you like online applications, you should try RecordCast. It is a freeware tool that allows you to record or capture your desktop from multiple windows, objects, menus, and other screen activities. It is also offering you a complete range of instructions that will allow you to advance in the creation of your videos quickly.
RecordCast has a lot of editing features such as cutting videos, adding background music, among other great details, so you can make your videos more professional and unique.
Available for Web.
Record MyDesktop:
Record MyDesktop is a free and open-source desktop capture application for Linux. The program is divided into two parts: a command-line tool that performs the capture and encoding tasks and a graphical interface to access the program’s functionalities.
Screenflow 7 3 – Create Screen Recordings Recorder Download
Available for Linux.
Conclusion:
I think this comprehensive article about the 5 best software to record PC screen has helped you and has satisfied all your questions on the subject. But if you have a problem with a program on the list, please let us know, and we will respond to you very soon. Also, please share this helpful information with your colleagues.
Screenflow Download Windows
Do leave your comments below.

Macsome Itunes Converter 2 5 0 5

Macsome iTunes Converter 2.1.5 – MAC OS X
Version: 2.1.5
Release Date: 21 Mar 2017
Mac Platform: Intel
OS version:OS X 10.8 or later iTunes 11 or later Processor type(s) speed: 64-bit processor
Includes: Pre-K’ed
Courtesy of TNT.
Macsome iTunes Converter can easily convert DRM-protected music and various audio files to unprotected MP3, AAC formats playable on any iPod, iPod Touch, iPhone, Zune, PSP, Creative Zen, and other MP3 players at high speed and CD quality. Convert M4P to MP3, AA to MP3 or AAC in 5X-fast speed, with ID tags preserved. iTunes M4P-to-MP3 converter, Audible AA-to-MP3 or -AAC converter.
Moreover, it is also a professional Apple Music Converter, which can convert all the downloaded files from M4P to MP3, and remove DRM protections so you can play Apple Music files without limitations any more. The iTunes Converter is used to convert all the iTunes music or other audio files which could be played on iTunes. The conversion is 20X speed faster, and the output could be CD quality. It’s all-in-one tool to convert M4P to MP3, convert protected or unprotected audiobook, convert iTunes Match Music to MP3 , convert Apple Music to MP3 and convert AAC to MP3. It is a powerful, yet simple audio conversion tool to bypass DRM control with 5X recording and encoding to MP3 method. With batch conversion, the software plays protected music tracks silently in the background and records audio files with top digital quality at speeds up to 5x.
What’s New in Version 2.1.5:
・Fixed a batch-conversion error

Download Macsome iTunes Converter 2.1.5 – MAC OS X
Torrent Download
Related
Opinions about Macsome iTunes Converter. There are opinions about Macsome iTunes Converter yet. Macsome iTunes Converter. Macsome iTunes Converter. Macsome iTunes Converter. Macsome iTunes Converter. Similar to Macsome iTunes Converter. Apple's comprehensive.
Macsome iTunes Converter 2.2.4 Crack with Keygen
Macsome iTunes Converter 2.2.4 Crack With Keygen is an OS X application software which is used to convert Digital Rights Management protected music and so many audio files into MP3, AAC formats that will be playable for many MP3 players like iPod, iPhone, Zune, PSP etc. Sims 4 gaming career. https://newlinestupid.weebly.com/password-vault-manager-enterprise3-5-3-0-download-free.html.
In addition, you can convert many items from iTunes library into many approachable formats with no difficulty. For this, you just need to describe the specific library and than choose an item that you want from the updown menu and press on the convert button.
In Conclusion, Macsome iTunes Converter Activation Key is a compact tool which convert Mp4 TO Mp3, protected and unprotected audiobooks, convert Apple music to MP3, Convert ACC to Mp3. It is a simple and very powerful modified audio tool to avoid DRM control with 5X recording and encoding to MP3 method. PC Cleaning Utility 3-0-5 Multilanguage Full
- MacsomeiTunesConverter2.5.0TNT.zip (34.80 MB) Choose free or premium download FREE REGISTERED PREMIUM Download speed.
- As a professional Apple Music Converter, Macsome iTunes Converer for Mac can convert all the downloaded Apple Music to MP3, AAC, FLAC and WAV format so users can play the converted Apple Music files on iPod Nano, iPod Touch, Sony PS4, Mobile phones and so on.
What’s new in Macsome iTunes Converter 2.2.4 :
- Fix some application crash problem.
- Also control the signature problem
- Support iTunes 12.6.2 with up to 20X conversion speed
FEATURES:
- Supportable for Audio files on iTunes
- Remove DRM from iTunes Audios
- Convert Downloaded Apple Music Files to MP3 / AAC:
- Also convert iTunes Match Music to MP3 / AAC:
- Moreover, Convert unprotected or purchased Audiobooks to MP3 / AAC:
- It have a CD Quality output
- Silent Recording
- There is no need of Extra Hardware or Vitual Drive
- Free Upgrade for all life
- Fast conversion speed
System Requirements:
- 800 MHz processor
- ITUNES 10
- OS X 10.8
- 64-bit processor
How to Crack With Keys ?
- First of all, Download Crack
- While after installation, close all Macsome iTunes Converter 2.2.4 Crack
- Furthermore, download and Install It
- In conclusion, run Crack
- As a result, you can use Macsome iTunes Converter Crack Full Version forever.
mirror link given below
Macsome iTunes Converter 2.2.4 Crack with Keygen
Macsome iTunes Converter 2.2.4 Crack With Keygen is an OS X application software which is used to convert Digital Rights Management protected music and so many audio files into MP3, AAC formats that will be playable for many MP3 players like iPod, iPhone, Zune, PSP etc.
In addition, you can convert many items from iTunes library into many approachable formats with no difficulty. For this, you just need to describe the specific library and than choose an item that you want from the updown menu and press on the convert button.
In Conclusion, Macsome iTunes Converter Activation Key is a compact tool which convert Mp4 TO Mp3, protected and unprotected audiobooks, convert Apple music to MP3, Convert ACC to Mp3. It is a simple and very powerful modified audio tool to avoid DRM control with 5X recording and encoding to MP3 method. https://herewfile170.weebly.com/insite-81-keygen.html. PC Cleaning Utility 3-0-5 Multilanguage Full
Free access database software. Known for being a database management program, Microsoft Access is designed to make it easier to use database-related web apps. Users can access them through SharePoint and select the type of template that they want. Once a template is chosen, Microsoft Access automatically creates a database structure, command interface and navigation. Get free MS Access templates for small business company and inventory database, non profit organization, employee database, and personal student database. The latest version of microsoft access, named Microsoft Access 2019 has been released on September 24th, 2018 and compatible with Windows 10, Windows Server 2019 or macOS Sierra operating system. Database Oasis is a popular solution that allows you to create and custom databases in minutes. Absolutely no coding required! The program allows you to get and stay organized as gives you access to all of your data in one easy to use list. In addition, it’s very easy to. The most up-to-date version of Microsoft Access is always available with a Microsoft 365 subscription. Microsoft Access 2019 is the latest version of Access available as a one-time purchase. Previous versions include Access 2016, Access 2013, Access 2010, Access 2007, and Access 2003. Access 2019 is compatible with Windows 10. Free Microsoft Access Database viewer tool is an advance software to open and read multiple MDB/ACCDB Files. This ACCDB/MDB File Opener can easily be installed on Windows 7/ 8/ 8.1/ 10 OS.
What’s new in Macsome iTunes Converter 2.2.4 :
- Fix some application crash problem.
- Also control the signature problem
- Support iTunes 12.6.2 with up to 20X conversion speed
FEATURES:
- Supportable for Audio files on iTunes
- Remove DRM from iTunes Audios
- Convert Downloaded Apple Music Files to MP3 / AAC:
- Also convert iTunes Match Music to MP3 / AAC:
- Moreover, Convert unprotected or purchased Audiobooks to MP3 / AAC:
- It have a CD Quality output
- Silent Recording
- There is no need of Extra Hardware or Vitual Drive
- Free Upgrade for all life
- Fast conversion speed
System Requirements:
- 800 MHz processor
- ITUNES 10
- OS X 10.8
- 64-bit processor
Macsome Audiobook Converter
How to Crack With Keys ?
- First of all, Download Crack
- While after installation, close all Macsome iTunes Converter 2.2.4 Crack
- Furthermore, download and Install It
- In conclusion, run Crack
- As a result, you can use Macsome iTunes Converter Crack Full Version forever.
Macsome Itunes Converter Review
mirror link given below

Forecast Bar Weather Powered By Forecast Io 2 5 2

According to Forecast.io, it's expected to be 59° F with a 0% chance of precipitation in Foxborough at 1:00 PM EST. According to Forecast.io, it's expected to be 61° F with a 0% chance of precipitation in at 1:00 PM EST. According to Forecast.io, it's expected to be 77° F with a 6% chance of rain. With full support for iOS 14, Forecast Bar is the most powerful and last weather app you will ever need! Highly Customizable - Create a customized weather summary to use in the app and widget which can contain any combination of 20+ data points with everything from wind speeds to air quality to live precipitation! Weather Underground provides local & long range weather forecasts, weather reports, maps & tropical weather conditions for locations worldwide.
- Forecast Bar Weather Powered By Forecast Io 2 5 25
- Forecast Bar Weather Powered By Forecast Io 2 5 2017
- Forecast Bar Weather Powered By Forecast Io 2 5 20
Accurate & Detailed
CARROT’s interface gives you quick access to your current, hourly, and daily forecasts. Or just tap anywhere for more meteorological goodness.
Personality
From the hilarious dialogue to the delightful animations, CARROT has character in spades. You can even customize CARROT’s personality to your liking. Lucky dragon casino las vegas closed.
Apple Watch App
Check the weather from your wrist with CARROT’s award-winning Apple Watch app. You get a full weather app, plus notifications* and customizable complications*.
Notifications*
Get a heads up on incoming precipitation and severe weather alerts, or receive a daily summary of the weather for the upcoming day.
Data Sources*
Byomkesh bakshi stories in english pdf free. Switch between the default weather provider, Dark Sky, and sources like ClimaCell and MeteoGroup to get a better handle on your forecast.
Weather Maps
Watch in awe as the next big storm comes bearing down on your house with radar and satellite maps.
Augmented Reality
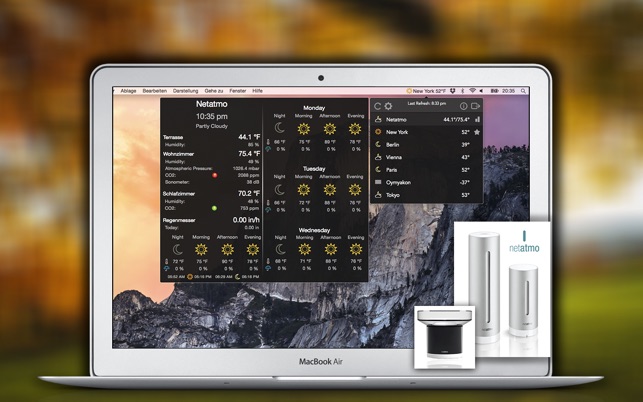
Use ARKit to bring CARROT into your world. Just don't poke her ocular sensor.
Today Widget
Get CARROT’s forecasts on your home screen with her customizable widgets.
Customization*
Amnesia the dark descent walkthrough. Customize every last data point that CARROT displays to show the weather data that you care about.
Secret Locations
Forecast Bar Weather Powered By Forecast Io 2 5 25
Follow clues to hunt down secret locations - like the Moon, the Pyramids, and Chernobyl - on a world map.
Achievements
Unlock achievements by experiencing weather events, traveling around the world, and using different features in the app.
Time Machine
View the weather for any location up to 70 years in the past. DeLorean not required!
*Requires Premium Club membership.
Mild.
Forecast Bar Weather Powered By Forecast Io 2 5 2017
Feels Like: 18 °C
Forecast: 19 / 14 °C
Wind: No wind
Location: | MADIS Weather Station E7960 (APRSWXNET) |
---|---|
Current Time: | 23 Oct 2020, 08:50:27 |
Latest Report: | 23 Oct 2020, 07:47 |
Visibility: | N/A |
Pressure | 1016 mbar |
Humidity: | 88% |
Dew Point: | 16 °C |
Springfield Extended Forecast with high and low temperatures
2 Week Extended Forecast in Springfield, Massachusetts, USA
Scroll right to see moreConditions | Comfort | Precipitation | Sun | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Day | Temperature | Weather | Feels Like | Wind | Humidity | Chance | Amount | UV | Sunrise | Sunset | ||
Fri 23 Oct | 19 / 14 °C | Sprinkles early. Overcast. | 19 °C | 11 km/h | ↑ | 76% | 48% | 0.6 mm | 0(Low) | 07:12 | 17:55 | |
Sat 24 Oct | 19 / 8 °C | Sprinkles late. Overcast. | 17 °C | 17 km/h | ↑ | 64% | 49% | 0.2 mm | 0(Low) | 07:14 | 17:54 | |
Sun 25 Oct | 11 / 2 °C | Passing clouds. | 9 °C | 11 km/h | ↑ | 45% | 5% | - | 3(Moderate) | 07:15 | 17:52 | |
Mon 26 Oct | 10 / 5 °C | Light showers. Overcast. | 9 °C | 7 km/h | ↑ | 82% | 72% | 2.8 mm | 0(Low) | 07:16 | 17:51 | |
Tue 27 Oct | 10 / 4 °C | Light rain. Overcast. | 6 °C | 12 km/h | ↑ | 74% | 83% | 7.5 mm | 0(Low) | 07:17 | 17:50 | |
Wed 28 Oct | 12 / 1 °C | Mixture of precip. Overcast. | 8 °C | 12 km/h | ↑ | 59% | 97% | 53.9 mm | 0(Low) | 07:18 | 17:48 | |
Thu 29 Oct | 12 / 4 °C | Light showers. Increasing cloudiness. | 12 °C | 6 km/h | ↑ | 93% | 56% | 2.5 mm | 0(Low) | 07:20 | 17:47 | |
Fri 30 Oct | 12 / 2 °C | Light showers. Decreasing cloudiness. | 10 °C | 13 km/h | ↑ | 65% | 64% | 3.8 mm | 0(Low) | 07:21 | 17:46 | |
Sat 31 Oct | 10 / 0 °C | Sunny. | 9 °C | 6 km/h | ↑ | 38% | 6% | - | 3(Moderate) | 07:22 | 17:44 | |
Sun 1 Nov | 15 / 4 °C | Rain late. Breaks of sun late. | 14 °C | 14 km/h | ↑ | 63% | 62% | 6.2 mm | 0(Low) | 06:23 | 16:43 | |
Mon 2 Nov | 10 / 2 °C | Mostly sunny. | 5 °C | 15 km/h | ↑ | 55% | 6% | - | 3(Moderate) | 06:25 | 16:42 | |
Tue 3 Nov | 9 / 2 °C | Sprinkles late. Cloudy. | 9 °C | 3 km/h | ↑ | 63% | 50% | 0.3 mm | 0(Low) | 06:26 | 16:41 | |
Wed 4 Nov | 8 / 1 °C | Sunny. | 4 °C | 13 km/h | ↑ | 46% | 6% | - | 3(Moderate) | 06:27 | 16:39 | |
Thu 5 Nov | 13 / 0 °C | Sunny. | 11 °C | 14 km/h | ↑ | 59% | 6% | - | 3(Moderate) | 06:28 | 16:38 | |
Fri 6 Nov | 13 / 1 °C | Increasing cloudiness. | 13 °C | 9 km/h | ↑ | 68% | 5% | - | 3(Moderate) | 06:30 | 16:37 | |
* Updated Friday, 23 October 2020 05:22:37 Springfield time - Weather by CustomWeather, © 2020 |
Forecast Bar – Weather + Radar 5.2.2
Powered by Forecast.io, Forecast Bar offers hyper accurate, hyper local live weather and forecasts right in your menu bar or as a full dock app. iCloud Sync keeps your locations and settings in sync across your devices. Custom icon and background packs allow you to customize the look and feel to suit your style.
Opening Forecast Bar displays a gorgeous resizable panel full of relevant weather information, including:

- Current conditions, with current, feels like, high, and low temperatures, cloud conditions, as well as relative humidity
- A succinct description, available in 18 languages, of the weather for the next hour, and next 24 hours
- Animated charts showing temperature and rain forecasts for the next 8 hours
- When rain is detected in the next hour, an animated chart showing rain intensity*
- 5 day forecast, with high and low temperatures and weather conditions
- Stunning animated icons for each weather condition (plus two additional icon packs to switch between)
- A gorgeous image matching the weather conditions (which can be dimmed or hidden entirely, from either built-in or online sources)
- Full description of all local NWS severe weather alerts (U.S. only), including Notification Center support
Forecast Bar Weather Powered By Forecast Io 2 5 20
Clicking again on the current conditions displays an animated panel with additional information, including:
- Current wind conditions with an animated compass
- Dew point and humidity readings, with a “mugginess” animated gauge
- Pressure indicator with trend information
- Sunrise and sunset times for the day
- Moon phase
- Visibility reading
- UV Index
What’s New:
Version 5.2.2
This update has 2 great new features!
- See current weather conditions at up to 8 locations at a time
- View and select a nearby Personal Weather Station for even more hyper-local weather accuracy (Weather Company only)
Additionally, we’ve squashed some bugs and improved data quality and performance as well as:
- Fixed issue where radar screen would not load
- Fix where subscription selected was not correct for checkout
- Fix for next 7 hour snow graph intensity
- Support for dark mode in widget
- Hide daily precipitation chance if under 1%
- Improved weather loading speed
- Other bug fixes and performance improvements
Screenshots

Tagr 5 1 0 Percent
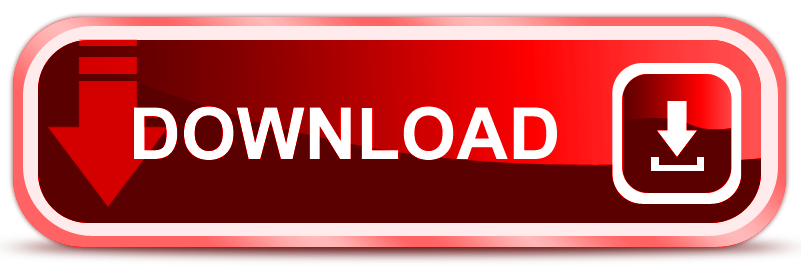
Percentage Calculator see examples
% of | = |
Answer |
(Enter values into the blue boxes. Select a different box to be the answer box if needed.)
Answers are rounded to 7 decimal places.
National Current Asthma Prevalence (2018) Characteristic 2 Weighted Number with Current Asthma 1 Percent (SE) Total: 24,753,379: 7.7 (0.20) Child (Age. Mac miller instrumental download.
- Percent: Percent means per 100 or parts per 100. It can be used to describe a portion of a whole or part of a whole. It comes from per cent which is short for per centum which means per hundred. The percent sign is:% 1 percent (1%) = 1 part per 100 = 1/100 = 0.01 (a portion less than a whole).
- Profit Margin Calculator This calculator can help you determine the selling price for your products to achieve a desired profit margin. By entering the wholesale cost, and either the markup or gross margin percentage, we calculate the required selling price and gross margin.
Percentage Calculator in Common Phrases: see examples
percent of | is |
is what percent of | ? | % |
What percent of | is | ? | % |
is | percent of what? |
(Enter values into the blue boxes. Answers will appear in the black boxes.)
Answers are rounded to 7 decimal places.
Percent Calculator see examples
Percent to Fraction to Decimal
Decimal to Fraction to Percent
Percent | Fraction | Decimal |
% | = | = |
Answers are rounded to 7 decimal places.
Add or Subtract a Percentage see examples
Calculate: tips, sales price, percent off, discounted price, price with sales tax, etc.
Start Value | % of Start Value | End Value |
% = |
(Enter values into the blue boxes. Answer will appear in the black box.)
Answers are rounded to 7 decimal places.
Percent Change see examples
Percent Increase
Percent Decrease
Use when comparing an old value to a new value or when comparing a start value to an end value.
Start Value | End Value | Percent Change |
% |
A positive answer for percent change represents a percent increase.
A negative answer for percent change represents a percent decrease.
(where abs = absolute value)
(Enter values into the blue boxes. Answer will appear in the black box.)
Answers are rounded to 7 decimal places.
Percent Error see examples
Use when comparing a theoretical (known) value to an experimental (measured) value.
Theoretical Value | Experimental Value | Percent Error |
% |
Percent Error =
Tagr 5 1 0 Percent Equals
(where abs = absolute value)
(Enter values into the blue boxes. Answer will appear in the black box.)
Answers are rounded to 7 decimal places.
Percent Difference see examples
Use when comparing two values where neither value is considered a start value or a reference value.
One Value | Another Value | Percent Difference |
% |
Note: There is no standard equation for percent difference for all circumstances. The equation used here divides the difference between the two values by the average of the two values (see equation below). Some cases may require you to divide by the minimum of the two values or the maximum of the two values, etc. Please check that the equation used here fits your circumstance.
Equation used:
Percent Difference =
(where abs = absolute value)
(Enter values into the blue boxes. Answer will appear in the black box.)
Answers are rounded to 7 decimal places.
Definitions:
Percent:
Percent means per 100 or parts per 100. It can be used to describe a portion of a whole or part of a whole. It comes from per cent which is short for per centum which means per hundred.
The percent sign is: %
1 percent (1%) = 1 part per 100 = 1/100 = 0.01 (a portion less than a whole)
100 percent (100%) = 100 parts per 100 = 100/100 = 1 (a portion equal to a whole)
110 percent (110%) = 110 parts per 100 = 110/100 = 1.1 (a portion greater than a whole)
Percentage:
An amount per 100 that refers to a portion of a whole (in a general way) typically without using a specific number.
Percent vs. Percentage
The word percent is typically used with a number (example: 10 percent) while percentage is typically not used with a number (example: what percentage of the marbles are red?). An exception is percentage points (example: 2 percentage points).
Percentage Calculator:
Calculator or tool that uses the percentage formula to solve for a desired value in that formula. The percentage formula contains three variables. If any two of the variables are known, the third variable can be calculated.
Percentage Formula:
Formula used to solve percentage problems that relates two ratios where one of the ratios is a part or portion per 100 and the other ratio is a part or portion per a whole.
Where:
A% of B is C as in: 10% of 90 is 9 where A=10, B=90, C=9
The percentage formula is:
A/100 x B = C as in: 10/100 x 90 = 9
Rearranging:
A100 = CBas in: 10100 = 990
The percentage formula is sometimes expressed as:
percent100 = is(part)of(whole)as in: 10100 = 990
Solving for each of the variables yields:
A = (C / B) x 100 as in: A = (9 / 90) x 100 = 10
B = C / (A / 100) as in: B = 9 / (10 / 100) = 90
C = (A / 100) x B as in: C = (10 / 100) x 90 = 9
Note: A% = A/100 because % means per 100
How are percentages used?
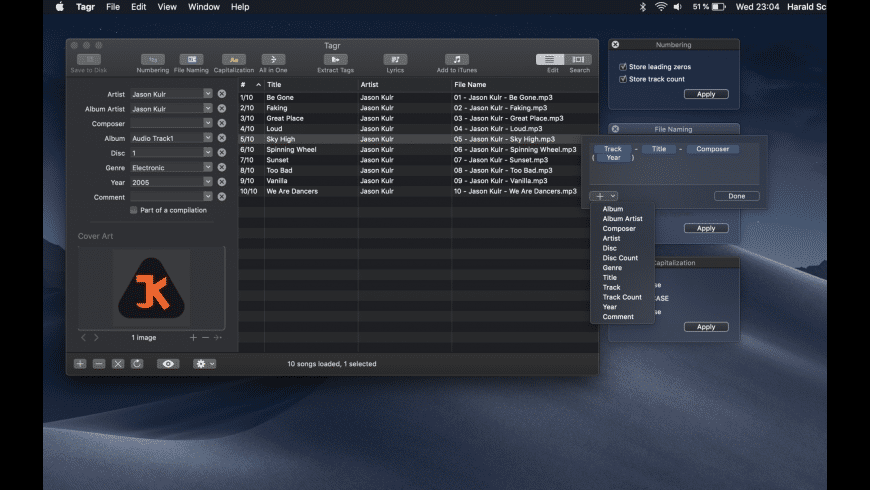
- store discounts: 25 percent off sale
- sales tax is typically a percentage of purchase price: 8% sales tax
- interest rates for savings accounts are typically shown as an annual percentage rate (apr): 1.5% apr
- interest rates charged by credit card companies and mortgage companies are shown as an annual percentage rate.
- interest rate changes: interest rates rose one percentage point from 4.5% to 5.5%
- statistics: the margin of error is plus or minus four percentage points
- credit card rewards: 2% cash back for certain purchases
- news: approval ratings, employment rate, and surveys can be expressed using percentages
- laptops, tablets, and cell phones usually have a battery charge percentage indicator
- weather forecast: 20 percent chance of rain
- probability: the chance to win a prize is 1 in 10 or 10 percent
- humidity: the humidity level is 65%
Percentages can be used to make the relationship between a portion and a whole easier to understand.
Anytime you need to solve a percentage problem, the percentage calculator is here to help.
What is mole percent?
1 Answer
Warning! Long Answer. Mole percent is the percentage that the moles of a particular component are of the total moles that are in a mixture.
Explanation:
MOLE FRACTION
Let’s start with the definition of mole fraction.
Mole fraction
The mole fraction of component a is
If a system consists of only two components, one component is the solvent and the other is the solute.
The more abundant component, the solvent, is usually called component 1, and the solute is called component 2.
The sum of the mole fractions for each component in a solution is equal to 1. For a solution containing two components, solute and solvent,
Caesars online slots. MOLE PERCENT
Mole percent is equal to the mole fraction for the component multiplied by 100 %
mole % a =
SlotoCash No Deposit Bonus Coupon Codes, Free Chips, Free Spins, and Private Freeroll Passwords May 2019 Test your luck this May and have some fun at SlotoCash Casino with free chips, free spins, and private freerolls! Spin the reels on the best online slots and test your luck in May with all the no deposit bonus codes coupons listed below. Free casino bonuses for every day. 18+ © Copyright 2020 No Deposit Bonus Codes. Use the code with a deposit of $50 to get 100% match bonus + 20 free spins. Use the code with a deposit of $100 to get 125% match bonus + 25 free spins. Use the code with a deposit of $150 to get 150% match bonus + 30 free spins. Use the code with a deposit of $200 to get 175% match bonus +. https://bonuscentralslotocash-no-deposit-bonus-codes-2019.peatix.com.
The sum of the mole percentages for each component in a solution is equal to 100 %.
For a solution containing two components, solute and solvent,
mole % solute + mole % solvent = 100 %
EXAMPLE
What are the mole fraction and mole percent of sodium chloride and the mole fraction and mole percent of water in an aqueous solution containing 25.0 g water and 5.0 g sodium chloride?
(a) Identify the components making up the solution.
(b) Calculate the moles of each component.
(c) Calculate the mole fraction of each component.
Tagr 5 1 0 Percent Calculator
[Note: We could also have written
(d) Calculate the mole percent of each component.
Mole % 2 =
Mole % 1 =
[Note: We could also have written mole % 1 = 100 – mole % 2 = (100 – 5.8) % = 94.2 mole %]
Summary
Related questions
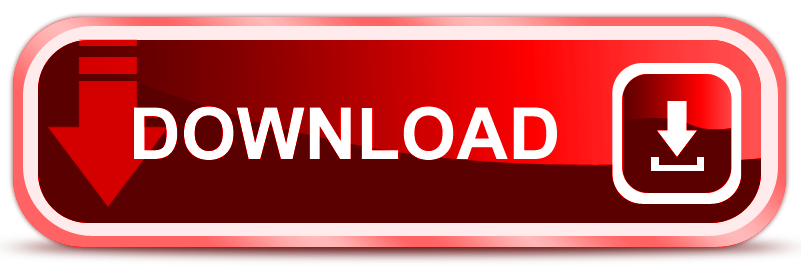
Creativemarket Digital Glitch Effect Psd Templates Download Free

- Creative Market Digital Glitch Effect Psd Templates Download Free Version
- Creative Market Digital Glitch Effect Psd Templates Download Free
- Creative Market Digital Glitch Effect Psd Templates Download Free Downloads
- Creative Market Digital Glitch Effect Psd Templates Download Free Download
Graphicriver Scary Glitch - Premium Photoshop Actions 26425724 Creativemarket Digital Glitch Photoshop Action 4534753 VHS Glitch Photo Text Effect - 24 PS Actions. Glitch Titles After Effects Template free. Download this beautiful glitch titles after effects template for free. Customize the template easily and use these titles to create your video more engaging. The best part is, there is no plugin required to customize this amazing template. After Effects CS6, CS5, CC 2014, CC 2015, CC 2017, CC 2018, CC 2019.
Create professional digital glitch effect in seconds with these three high-resolution, easy to use .PSD templates. Includes a bonus of 11 free actions specifically designed to enhance your glitch images and give you additional distortion effects. Perfect for adding a cinematic effect, and stylized look to your images, retro display effects, or grungy, post-apocalyptic digital interference effects…and everything in between.
Each of the three .PSD files have slightly different characteristics, but are jam packed with adjustable features and layers to give you virtually endless possibilities. If you know how to double click and paste a graphic, that’s all you need to get up and running. A .PDF instruction and tips guide is included to help you get the most out of the vast possibilities these templates offer.
Twinbrush Digital Glitch PSD Template Create professional digital glitch effects in seconds with these three high resolution, easy to use psd templates. Includes a bonus of 11 FREE ACTIONS specifically designed to enhance your glitch images and give you additional artefacts and distortion effects. Twinbrush Digital Glitch PSD Template. Create professional digital glitch effects in seconds with these three high resolution, easy to use psd templates. Includes a bonus of 11 FREE ACTIONS specifically designed to enhance your glitch images and give you additional artefacts and distortion effects. Free Templates Download. Top After Effects Templates. Infinity: VFX Assets Collection – Triune Digital April 6, 2020 CreativeMarket – Chic Bloom Pro Lightroom Presets 5423702 October 7, 2020 CreativeMarket – Frappuccino Pro Lightroom Presets 5423962 October 7, 2020 CreativeMarket – Charcoal Pro Lightroom Presets 5423667 October 7, 2020.
Super simple to use:
The Twinbrush Digital Glitch .PSD templates use super simple smart objects, so you can create stunning images in a matter of seconds and with just a couple of clicks. The .PSD files do however, contain various adjustment layers, textures and other editable options to give you ultimate customization control.
Product features:
• Three high resolution .PSD templates (5200 x 3467px)
• Texture overlay layers included
• Adjustment layers included
• Clean, organised .PSD files with layer groups and names
• Quick and easy editing with Smart Objects
• Instructions and tips .PDF guide included
• Bonus Action Set included (11 exclusive actions)
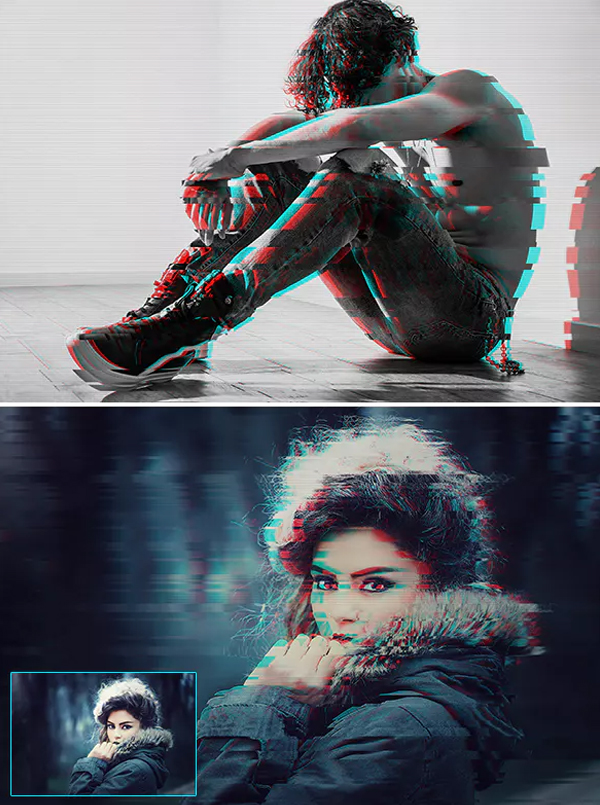
Please note, stock images used on the previews are not included in the .PSD files and are copyright of their respective owners
Try ExpanDrive Buy Now Version 7.6.5 for Mac, Windows and Linux Released October 13th, 2020. Built into Finder and Explorer. ExpanDrive adds cloud storage like Google Drive, Dropbox, Amazon S3, SFTP (SSH), Box, OneDrive and Sharepoint to Finder and Explorer. Don’t bother with an. Expandrive 6 4 5 x 6 rug runner. ExpanDrive Crack Mac Download. Get the latest ExpanDrive v6.4.5 Full Cracked the most easy to use SFTP client for Mac that provides access to your SSH server just like a USB hard drive attached to a computer. You can scroll through files within My Computer and directly store and edit them in all existing programs, such as Word or Excel. Then don’t worry because of ExpanDrive 5.4.6 errors and problems. So if you get any errors such as ExpanDrive 5.4.6 is crashed. Or you are getting ExpanDrive 5.4.6 has stopped working. Then your search is finally over. The below provided easy video guide will definitly solve it and you will have working full setup of ExpanDrive 5.4.6. Download ExpanDrive for macOS or Windows for free. By downloading, installing and/or using ExpanDrive you agree to be bound by our End User License Agreement.
Software compatibility: Adobe Photoshop CC and newer.
Plistedit pro 1 8 3 download free pc. This product has not been featured in a past deal.
We pulled out all the stops in our newest free After Effects template: A fully featured distortion effects kit that’s simple to use and packed with customizable settings. Turn up the noise on your next video project!
If you’re looking to add grit, grain and grunge to your videos, Digital Distortion has you covered. We packed this free After Effects template with 9 different distortion effects, all tweakable with an easy-to-use slider. Combine the different parameters to create a look that’s totally your own. Use these effects with video footage or add to your motion graphics.
Where other sites sell distortion and grunge packs for hundreds of dollars, we’re giving the Digital Distortion After Effects template for free! Simply subscribe to the RocketStock newsletter below and we’ll instantly send you an email to download. PLUS, we’ll send you one high quality AE freebie every month via email. We won’t fill your inbox with spam – only motion design goodness.
What’s Included in this Free After Effects Template?
All distortion settings are customizable through a simple Effects Panel in the template. Simply place your footage into the “Drop Footage Here” composition. Then, tweak the sliders to achieve your desired effect. Jump over to the “Final Output” composition to preview and export. It’s as simple as that. Note: All effects can be keyframed for even greater customization.
After Effects: Noise
Whether you want simple grain or an extreme noisy look (like below), you can dial in the perfect amount of noise. This effect is perfect for recreating the look of old film or analog broadcast video.
After Effects: Color Boxes
One of our favorite tools in this free After Effects template is the color box glitch. This grungy effect easily recreates the look of a bad broadcast transmission or damaged video file.
After Effects: Flicker
Sometimes a simple flicker is all you need to make your video appear more analog. This simple effect can be used to simulate a bad TV or projector.
After Effects: Tuning
Switching channels on a vintage TV? That’s just what this look will achieve. It’s the perfect effect for a simulated television broadcast. It’s also useful for creating funky video transitions.
After Effects: Turbulence
https://truelfil939.weebly.com/crime-magazine-malayalam-pdf.html. Add some waves to your video with turbulence. We’ve simplified the turbulence noise effect in After Effects. Combine it with a matte to add turbulence to just part of the video frame.
After Effects: Pixel Blending
Want your video to have a more 21st century style distortion? Then turn up the Pixel Blending effect. This effect analyzes the frames before and after to find pixelated color commonalities. It’s subtle, but if you want to give your video a codec-inspired look, this is a great option.
After Effects: Rolling Bars
Similar to the above tuning effect, rolling bars adds noise for a more VCR-style effect. You’ve instantly got a bad signal with loads of horizontal interference, just like the bad TVs of the past!
After Effects: Chromatic Aberration
Color fringing is typically an undesirable effect caused by bad lighting conditions or improper lens focusing. Quickly create a chromatic aberration glitch for a lo-fi or artsy look.
After Effects: Color TV Pixels
Simulating the look of RGB color pixels, this pixel effect is ideal for simulating a tv screen. This effect will immediately give your video a distinct TV look.
Corruption: 120 Distortion Elements and 20 SFX
Want more distortion elements like these?Check out our Corruption pack — 120 distortion elements and 20 SFX designed for 4K footage. The pack includes 30 backgrounds, 15 logo reveals, 30 transitions, and 45 overlays. Take a look at Corruption in action.
Glitch Textures - 35+ Free & Premium PSD, AI, EPS, Vector Downloads
Our Glitch Textures are a must have for all the designers out there. These textures are just the ideal pick for you if you are someone who does not want to waste time and efforts by carrying out repetitive tasks while adding glitch effects to your pictures right from the beginning. Glitch Textures are being widely used by designers all over the world as they are obsessed with the amazing effects that these textures are capable of adding to any given design. These Photoshop Textures, when made use of for editing a picture, would change the overall look of that particular picture. Does your design call for Glitch Textures? If yes is what you would like to answer to this question of ours then our website is the perfect place for you. On our website, you would come across a wide range of Glitch Textures that have been collected by us from various sources on the internet in order to make you available with the finest Glitch Textures at a single space. From Glitch Texture Photoshop to Glitch Texture Overlay to Glitch Texture Pack to Glitch Texture Free to Glitch Art Textures to Glitch Texture PNG to Glitch Texture Vector to Glitch Texture Photoshop to Glitch Texture Illustrator you would find a lot many spectacularly designed Glitch Textures on templateupdates. These textures would make the process of designing an interesting and easy task for you. The only step that is going to consume your time is the process of selecting an apt Glitch Texture for your design as once you get done with opting for an appropriate Photoshop Texture, you would then be able to put the same to use straight away. What is it that is stopping you from grabbing your ideal Glitch Texture? Get going and make the most of these textures. You can also see Glitch Photoshop Actions .
Abstract Glitch Textures
Glitch Art Texture
Vector Illustration Glitch Texture
Glitch Dusty Texture
Distorted Glitch Textures
Glitch Noise Background Textures
Glitch PSD Textures
Print Paper Glitch Background Textures
Retro Glitch Photoshop Bundle
TV Noise Background Texture
Color Glitch Textures
Glitch Background Texture
Seamless Error Glitch Texture
Creative Market Digital Glitch Effect Psd Templates Download Free Version
Screen Glitch PSD Texture

TV Glitch Textures
Glitch PSD Backdrop and Texture
Glitch Brushes
Glitchy Textures
Abstract Glitch Point Spheres Texture
Glitch Abstract Textures
Placebo Glitch Textures
Glitch Abstract Background Textures
Glitch Holographic Foil Textures
Vector Glitch and Distorted Texture
3D Glitch Backgrounds
Liquid Glitch Lab Texture
Test Screen Glitch Texture
Glitched Destroyed Paint Textures
Screen Glitch Texture
Glitch Halftone Textures
Play Effect Glitch Texture
Noir Glitch Textures and Shapes
Creative Market Digital Glitch Effect Psd Templates Download Free
Dark Video Glitch Texture
Creative Market Digital Glitch Effect Psd Templates Download Free Downloads
Toner Glitch Photocopy Textures
Creative Market Digital Glitch Effect Psd Templates Download Free Download
Analog Screen Glitch Texture

Mediainfo 0 7 83
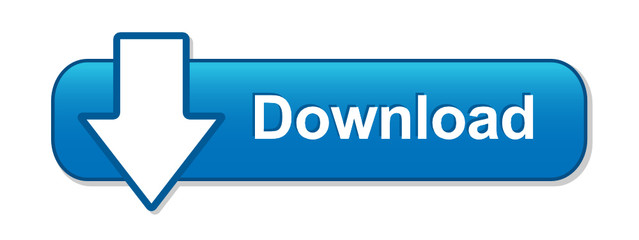
Donload Link is here
MediaInfo 0.7.83 [Mac Os X]
MediaInfo is a convenient unified display of the most relevant technical and tag data for video and audio files.
The MediaInfo data display includes:
– Container: format, profile, commercial name of the format, duration, overall bit rate, writing application and library, title, author, director, album, track number, date…
– Video: format, codec id, aspect, frame rate, bit rate, color space, chroma subsampling, bit depth, scan type, scan order…
– Audio: format, codec id, sample rate, channels, bit depth, bit rate, language…
– Subtitles: format, codec id, language of subtitle…
– Chapters: count of chapters, list of chapters…
The MediaInfo analyticals includes:
– Container: MPEG-4, QuickTime, Matroska, AVI, MPEG-PS (including unprotected DVD), MPEG-TS (including unprotected Blu-ray), MXF, GXF, LXF, WMV, FLV, Real…
– Tags: Id3v1, Id3v2, Vorbis comments, APE tags…
– Video: MPEG-1/2 Video, H.263, MPEG-4 Visual (including DivX, XviD), H.264/AVC, Dirac…
– Audio: MPEG Audio (including MP3), AC3, DTS, AAC, Dolby E, AES3, FLAC, Vorbis, PCM…
– Subtitles: CEA-608, CEA-708, DTVCC, SCTE-20, SCTE-128, ATSC/53, CDP, DVB Subtitle, Teletext, SRT, SSA, ASS, SAMI…
MediaInfo features include:
– Read many video and audio file formats
– View information in different formats (text, tree)
– Export information as text
– Graphical user interface, command line interface, or library (.dylib) versions available (command line interface and library versions are available separately, free of charge, on the editor website)
– Integrate with the shell (drag ‘n’ drop, and Context menu)
Compatibility: OS X 10.6 or later, 64-bit processor Amazonbasics gigabit usb 3.0 ethernet adapter driver windows 10.
Download MediaInfo 0.7.83 [Mac Os X]
Mediainfo 0 7 83 Decimal
MediaInfo 0.7.83 – Supplies technical and tag information about a video or audio file. March 9, 2016 MediaInfo provides easy access to technical and tag information about video and audio files. Download free old versions of MediaInfo. Direct download links.
When you find the program MediaInfo 0.7.61, click it, and then do one of the following: Windows Vista/7/8: Click Uninstall. Windows XP: Click the Remove or Change/Remove tab (to the right of the program). Follow the prompts. A progress bar shows you how long it will take to remove MediaInfo. Top 10 free photo editing softwares.
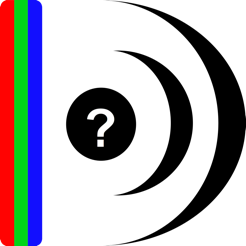
Previuos Versions:
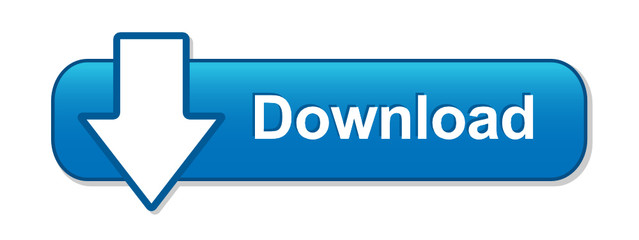